In this article we will see that how to create dynamic tabs.
For making dynamic tabs I will use Ajax Tab Container controls. I will create tabs dynamically, will add controls to each of the tabs dynamically and after that we will read the value of each control on some button click.
Step 1: Open visual studio and create a web site.
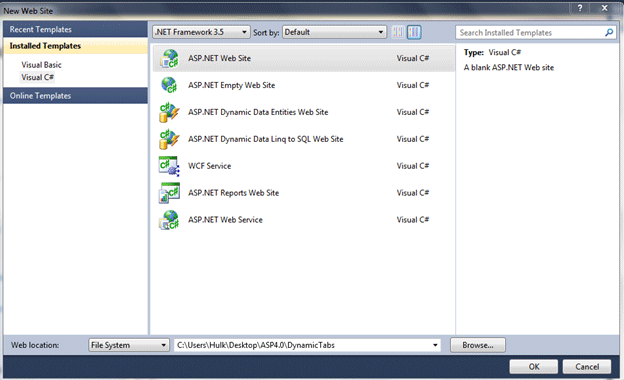
STEP 2: Add the refrence to AjaxToolkit.
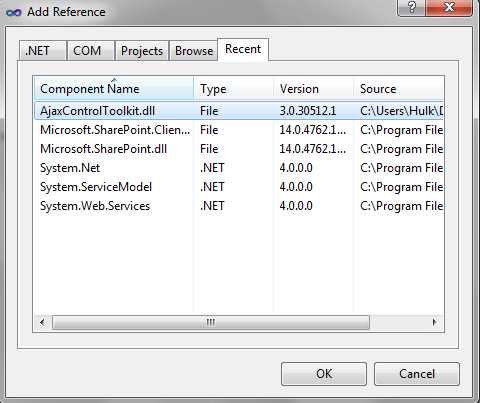
Now I Have Created One User control as AjaxTabUserControl.ascx as shown in below screenshot
Here is my AjaxTabUserControl.ascx Source Code:-
<%@ Control Language="C#" AutoEventWireup="true" CodeFile="AjaxTabUserControl.ascx.cs" Inherits="AjaxTabUserControl" %>
<asp:ScriptManager ID="ScriptManager1" runat="server">
</asp:ScriptManager>
<div>
<asp:PlaceHolder ID="PlaceHolder1" runat="server"></asp:PlaceHolder>
</div>
I have Taken one Sql server Database inside App_Data Folder as SwashAjaxTab.mdf and One Link To Sql Classes As DataClasses.dbml as shown in below figure:
Now I have Created Two Table As shown In Below Two Figure:-
Here Is My Code Behind Of My AjaxTabUserControl.ascx:-
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
using AjaxControlToolkit;
public partial class AjaxTabUserControl : System.Web.UI.UserControl
{
AjaxControlToolkit.TabContainer DynamicTab;
protected void Page_Load(object sender, EventArgs e)
{
DataClassesDataContext dc = new DataClassesDataContext();
var x = GetTabInfo();
for (int i = 0; i < x.Count(); i++)
{
Table tb1 = new Table();
TableRow tr1 = new TableRow();
TableCell tc1 = new TableCell();
DynamicTab.Tabs[i].Controls.Add(tb1);
var y = GetNewItemInfo(x[i].TabID);
DropDownList drplist = new DropDownList();
for (int j = 0; j < y.Count(); j++)
{
drplist.DataSource = y;
drplist.DataTextField = "NewItem1";
drplist.DataBind();
}
tc1.Controls.Add(drplist);
tr1.Cells.Add(tc1);
tb1.Rows.Add(tr1);
}
PlaceHolder1.Controls.Add(DynamicTab);
}
protected void Page_Init(object sender, EventArgs e)
{
try { createTab(); }
catch { }
}
private List<TabItem> GetTabInfo()
{
using (DataClassesDataContext context = new DataClassesDataContext())
{
return (from c in context.TabItems
select c).ToList();
}
}
private List<NewItem> GetNewItemInfo(int Tid)
{
using (DataClassesDataContext context = new DataClassesDataContext())
{
return (from n in context.NewItems where n.TabID==Tid
select n).ToList();
}
}
private void createTab()
{
DynamicTab = new AjaxControlToolkit.TabContainer();
DataClassesDataContext dc = new DataClassesDataContext();
var x = GetTabInfo();
for (int i = 0; i < x.Count(); i++)
{
TabPanel TbPnlCategry = new TabPanel();
TbPnlCategry.HeaderText = x[i].TabName;
TbPnlCategry.ID = x[i].TabID.ToString();
DynamicTab.Tabs.Add(TbPnlCategry);
}
}
}
Now I have Created One Aspx Page As Default2.aspx and Use that User Control.
Here is my Source Code:
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="Default2.aspx.cs" Inherits="Default2" %>
<%@ Register src="AjaxTabUserControl.ascx" tagname="AjaxTabUserControl" tagprefix="uc1" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title></title>
</head>
<body>
<form id="form1" runat="server">
<div>
<uc1:AjaxTabUserControl ID="AjaxTabUserControl1" runat="server" />
</div>
</form>
</body>
</html>
Here I have Enter Some Data Into My table as mentioned in below screen shot:
After Running The .aspx page We got The Result As :