Introduction
In my blog I am going to tell how to download files using a gridview in asp.net. I have used C# as my language. I am using Visual studio 2008 professional as my tool. If you wish you may use Visual studio 2005 as well. Also I am using MS SQL as my database server.
1. How to start
- First open visual studio.
- Then go to file->new->website

- Add a new website.
- I have named as download.
- I am saving it in my D drive in folder named as "Download".

2. Create a new database
- Add a new connection.
- Create a new database
- Create a table called download
- Create the table definitions as shown in the screen shot
Add connection

Create a database

Create a new table

Include table defintion

- downloadId - int with auto increment(as a primary key)
- fileName - varchar(50)
- fielPath - varchar(MAX)
Create a folder
- Create a folder to save some files
- It will be usefull for downloading example
Include some files inside the folder

Adding data to the table
- Open table data
- Give the path of the saved files in the folder "download"

3. Adding a gridview
- Drag an drop a gridview to your default page
- Then drag and drop a SQL data source to your page
Add grid view

4. Add sql datasource

The full view

configure datasource

Choose connectin string

Select the table and fields

Test the query

Choose datasource for Gridview

Remove fields
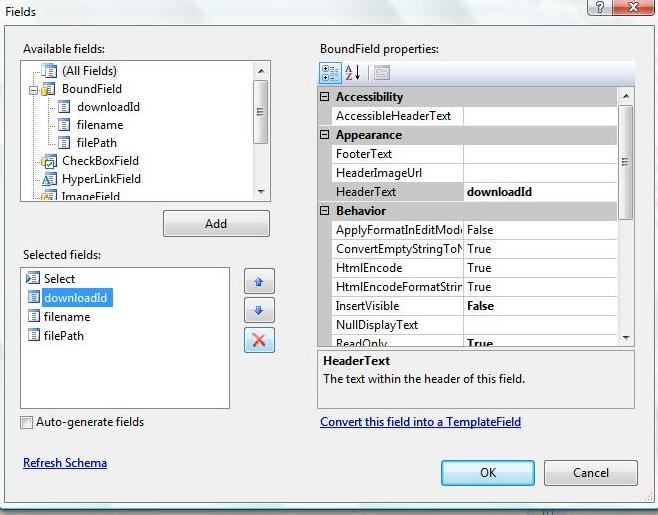
Change field names

View

Choose a format
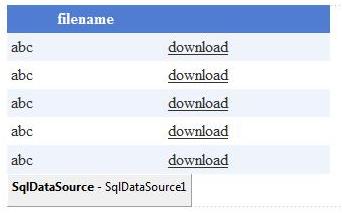
5. Create Appcode

Go to asp.net folder and choose appcode
Create a class called ConnectionManager

Code inside the ConnectionManager class
using System;
using System.Data;
using System.Configuration;
using System.Linq;
using System.Web;
using System.Web.Security;
using System.Web.UI;
using System.Web.UI.HtmlControls;
using System.Web.UI.WebControls;
using System.Web.UI.WebControls.WebParts;
using System.Xml.Linq;
using System.Data.SqlClient;
public class ConnectionManager
{
public static SqlConnection GetDatabaseConnection()
{
string connectString = "Data Source=VINO-PC\\SQLEXPRESS;Initial Catalog=download;Integrated Security=True";
SqlConnection connection=new SqlConnection(connectString);
connection.Open();
return connection;
}
Create another class called download

Code in download class(getting the selected file path)
using System;
using System.Data;
using System.Configuration;
using System.Linq;
using System.Web;
using System.Web.Security;
using System.Web.UI;
using System.Web.UI.HtmlControls;
using System.Web.UI.WebControls;
using System.Web.UI.WebControls.WebParts;
using System.Xml.Linq;
using System.Data.SqlClient;
public class download
{
public download()
{
}
public static DataSet GetresourcePath(int downloadId)
{
DataSet dataSet = new DataSet();
using (SqlConnection connection = ConnectionManager.GetDatabaseConnection())
{
string sql = "SELECT filePath FROM Download WHERE downloadId=@downloadId";
SqlCommand command = new SqlCommand(sql, connection);
command.Parameters.Add("@downloadId", SqlDbType.VarChar).Value = downloadId;
command.CommandType = CommandType.Text;
SqlDataAdapter dataAdapter = new SqlDataAdapter(command);
dataAdapter.Fill(dataSet, "filePath");
}
return dataSet;
}
}
Code behind for Default.aspx page(Default.aspx.cs)
using System;
using System.Configuration;
using System.Data;
using System.Linq;
using System.Web;
using System.Web.Security;
using System.Web.UI;
using System.Web.UI.HtmlControls;
using System.Web.UI.WebControls;
using System.Web.UI.WebControls.WebParts;
using System.Xml.Linq;
using System.Data.SqlClient;
using System.IO;
public partial class _Default : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
}
protected void GridView1_SelectedIndexChanged(object sender, EventArgs e)
{
try
{
int fileindex = (int)GridView1.SelectedValue;
string dbPath = null;
DataSet path = download.GetresourcePath(fileindex);
foreach (DataRow row in path.Tables["filePath"].Rows)
{
dbPath = string.Format("{0}", row["filePath"]);
}
string filePath = @dbPath;
string fileName = Path.GetFileName(filePath);
System.IO.Stream stream = null;
stream = new FileStream(filePath, System.IO.FileMode.Open, System.IO.FileAccess.Read, System.IO.FileShare.Read);
long bytesToRead = stream.Length;
Response.ContentType = "application/octet-stream";
Response.AddHeader("content-Disposition", "attachment; filename=" + fileName);
while (bytesToRead > 0)
{
if (Response.IsClientConnected)
{
byte[] buffer = new Byte[10000];
int length = stream.Read(buffer, 0, 10000);
Response.OutputStream.Write(buffer, 0, length);
Response.Flush();
bytesToRead = bytesToRead - length;
}
else
{
bytesToRead = -1;
}
}
}
catch (Exception ex)
{
}
}
}
Code behind detail
- To get the GridView1_SelectedIndexChanged method double click on the download link in the gridview.
- So it automatically create the method
- You may type the code I have given.
6. Now run and you may download your files
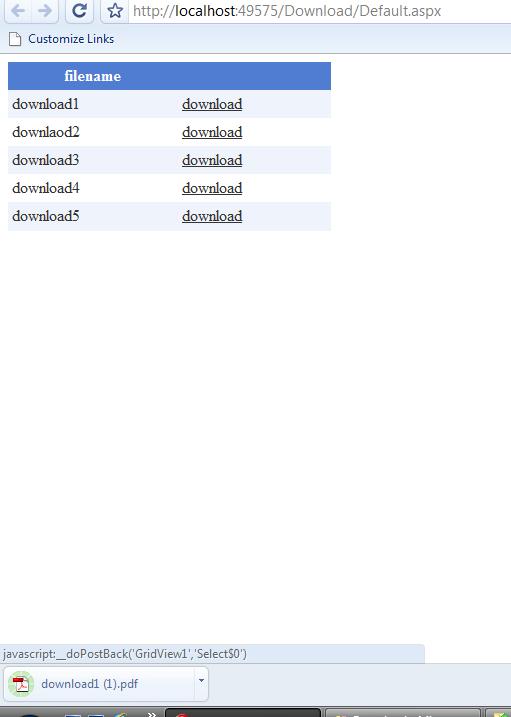
If you are facing trouble then you can try this following link also
Thanks Shibashish Mohanty
No comments:
Post a Comment
Please don't spam, spam comments is not allowed here.